Installing Ruby on Rails Edge with Bundler
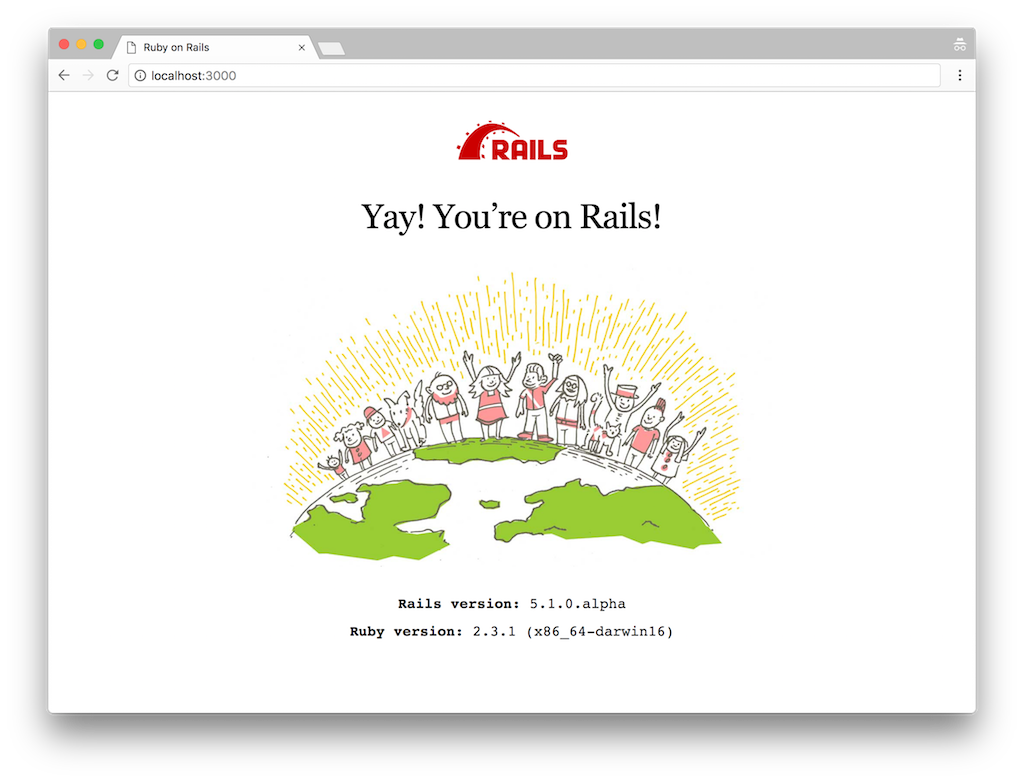
Sometimes you want to check out the latest commits to Ruby on Rails before it's made into an official release. Generally installing Rails is as simple as gem install rails
but getting the latest code committed to the rails/rails master branch is a little more complicated. Let's get you making new apps with the newest of the new.
Before going any further, make sure Ruby and Bundler are installed.
$ ruby --version
ruby 2.3.1p112 (2016-04-26 revision 54768) [x86_64-darwin16]
$ bundler --version
Bundler version 1.14.3
If either of those have an error, see installing Ruby or getting started with Bundler
Now make the directory where the Rails app will live. Typically this would automatically be handled with rails new blog
but in this instance manually make it. Inside of the blog
directory make a Gemfile
file.
mkdir blog
cd blog
touch Gemfile
Save the following few lines of code to the Gemfile
with your editor of choice (Atom is excellent if you need somewhere to start). This will tell bundler to use RubyGems.org as a source for resolving gems and then to install the gem rails
via Git directly from the source on GitHub.
source 'https://rubygems.org'
gem 'rails', git: 'https://github.com/rails/rails.git'
Now tell bundler to actually install the requested gems.
$ bundler install
Fetching https://github.com/rails/rails.git
Fetching gem metadata from https://rubygems.org/.............
Fetching version metadata from https://rubygems.org/.
Resolving dependencies...
If you get a compatibility error from bundler, you will have to add additional requirements to the Gemfile
.
Bundler could not find compatible versions for gem "arel":
In Gemfile:
rails was resolved to 5.1.0.alpha, which depends on
activerecord (= 5.1.0.alpha) was resolved to 5.1.0.alpha, which depends on
arel (~> 8.0)
Could not find gem 'arel (~> 8.0)', which is required by gem 'activerecord (= 5.1.0.alpha)', in any of the sources.
Go find the source repo for arel
. RubyGems.org hosts gems at https://rubygems.org/gems/<name>
so we'll find arel
at https://rubygems.org/gems/arel. Most gems will have a Source Code
link that links to online hosted source code on a site like GitHub. For arel
you'll end up at https://github.com/rails/arel
which has a Clone or download
button. You'll want to grab the HTTPS URL from this and add it to the Gemfile
.
source 'https://rubygems.org'
gem 'rails', git: 'https://github.com/rails/rails.git'
gem 'arel', git: 'https://github.com/rails/arel.git'
Run bundler install
again and repeat the previous step until you see a success message.
$ bundle install
Fetching https://github.com/rails/rails.git
Fetching https://github.com/rails/arel.git
Fetching gem metadata from https://rubygems.org/.............
Fetching version metadata from https://rubygems.org/.
Resolving dependencies...
[snipped]
Bundle complete! 2 Gemfile dependencies, 38 gems now installed.
Use `bundle show [gemname]` to see where a bundled gem is installed.
Within the blog
directory you now have access to the latest Rails code available.
$ rails --version
Rails 5.1.0.alpha
We are now ready to run the Rails app setup command.
rail new . --dev
The .
tells rails to use the current directory (blog
) instead of creating a new directory which is more typical. The --dev
will tell rails to use the latest development version you just installed. Otherwise Bundler will try and find gem version on RubyGems.org that are not available yet.
$ rails new . --dev
exist
create README.md
create Rakefile
create config.ru
create .gitignore
conflict Gemfile
Overwrite /Users/abraham/dev/rails-mdc-web/blog/Gemfile? (enter "h" for help) [Ynaqdh]
When prompted, you'll want to confirm (Y
) that you want to overwrite the Gemfile
.
You should now see a success message that the Rails app was created.
[snipped]
Bundle complete! 13 Gemfile dependencies, 59 gems now installed.
Use `bundle show [gemname]` to see where a bundled gem is installed.
One final edit to the Gemfile
. The rails/rails
dependency is currently pointed at your local filesystem version of Rails:
gem 'rails', path: '/Users/abraham/.rvm/gems/ruby-2.3.1/bundler/gems/rails-898d8787198f'
You need to update it to point at GitHub again.
gem 'rails', github: 'rails/rails'
Follow with one more Bundle to update the Gemfile.lock
with the just made Gemfile
change.
$ bundle install
[snipped]
Bundle complete! 13 Gemfile dependencies, 59 gems now installed.
Use `bundle show [gemname]` to see where a bundled gem is installed.
Now would be a good time to create an initial Git commit.
$ git add .
$ git commit -m 'Initial clean Edge Rails'
[master (root-commit) 132ded5] test
73 files changed, 1153 insertions(+)
create mode 100644 .gitignore
create mode 100644 Gemfile
create mode 100644 Gemfile.lock
[snipped]
You are now all ready to go.
$ rails server
=> Booting Puma
=> Rails 5.1.0.alpha application starting in development on http://localhost:3000
=> Run `rails server -h` for more startup options
Puma starting in single mode...
- Version 3.7.0 (ruby 2.3.1-p112), codename: Snowy Sagebrush
- Min threads: 5, max threads: 5
- Environment: development
- Listening on tcp://0.0.0.0:3000
Use Ctrl-C to stop
A warning though: Rails Edge is often fresh code that can contain bugs or regressions. You do not want to build production apps on it.